Hive Database Integration in Flutter – Beginner’s Guide
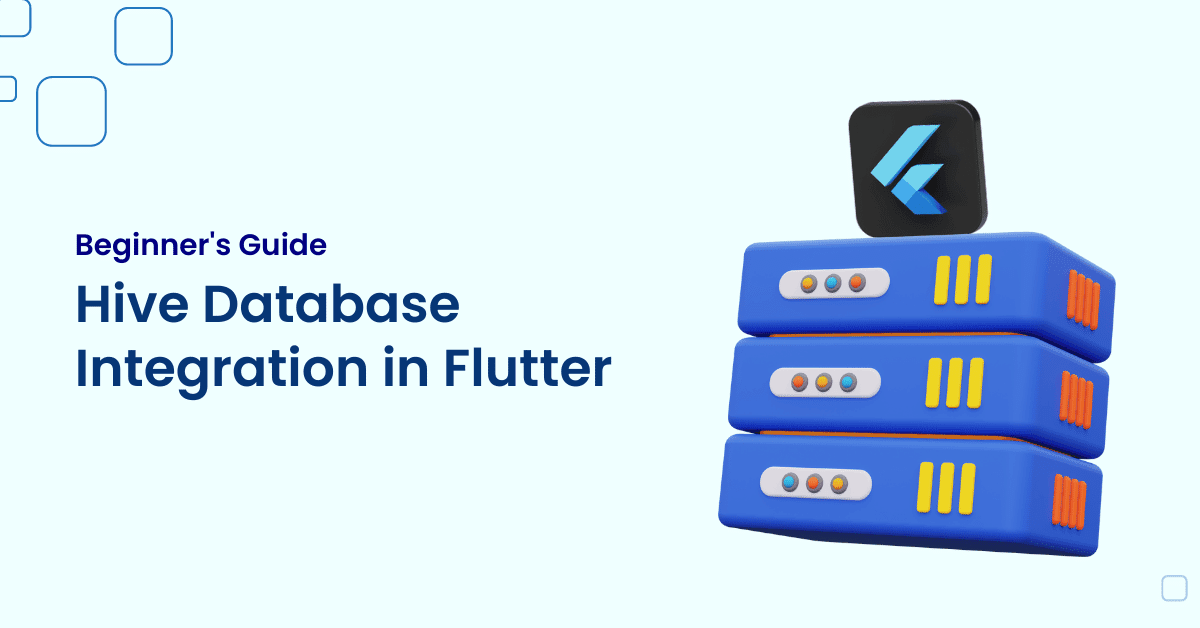
Introduction
Hive is a lightweight and fast NoSQL database that is easy to use and integrates well with Flutter. Hive stores data in key-value pairs and is optimised for fast read-and-write operations. It can be used to store data locally on the device as well as on the cloud. In this blog, we will be discussing how to use Hive Database Integration in Flutter to store and retrieve data from a local database.
Setup
Before we can use Hive in our Flutter application, we need to add the necessary dependencies to our pubspec.yaml file.
Open your pubspec.yaml file and add the following dependencies:
dependencies:
hive: ^2.2.3
hive_flutter: ^1.1.0
path_provider: ^2.0.13
Once you have added these dependencies, run flutter pub get to download and install them.
Getting started
Now that we have set up the dependencies, we can start using Hive in our Flutter application. The first step is to initialize Hive by calling Hive.initFlutter() in our main() function. We also need to register any custom adapters that we want to use with Hive.
void main() async {
await Hive.initFlutter();
Hive.registerAdapter(MyModelAdapter());
runApp(MyApp());
}
In the above code, we are registering an adapter for a custom model called MyModel. This is necessary so that Hive knows how to serialise and deserialize our data.
Creating a Hive box
In Hive, data is stored in boxes. A box is similar to a table in a traditional SQL database. We can create a new box by calling Hive.openBox().
final box = await Hive.openBox('myBox');
In the above code, we are creating a new box called myBox. We can now use this box to store and retrieve data.
Storing data
To store data in our box, we need to create a new instance of our model and add it to the box.
final myModel = MyModel(name: 'John', age: 25);
await box.add(myModel);
In the above code, we are creating a new instance of MyModel with a name of ‘John’ and an age of 25. We are then adding this instance to our box.
Retrieving data
To retrieve data from our box, we can call box.get() or box.values.
final myModel = box.get(0);
final myModels = box.values.toList();
In the above code, we are retrieving the first instance of MyModel from our box and storing it in a variable called myModel. We are also retrieving all instances of MyModel from our box and storing them in a list called myModels.
Updating data
To update data in our box, we can retrieve an instance from the box, modify it, and then save it back to the box.
final myModel = box.get(0);
myModel.age = 26;
await box.put(0, myModel);
In the above code, we are retrieving the first instance of MyModel from our box, updating its age to 26, and then saving it back to the box.
Deleting data
To delete data from our box, we can call box.delete() or box.deleteAt().
await box.delete(0);
await box.deleteAll();
In the above code, we are deleting the first instance of MyModel from our box using delete() method. We can also delete all the data from the box using deleteAll() method.
Closing the box
Once we are done using a box, we should close it to free up resources.
await box.close();
In the above code, we are closing the box we created earlier.
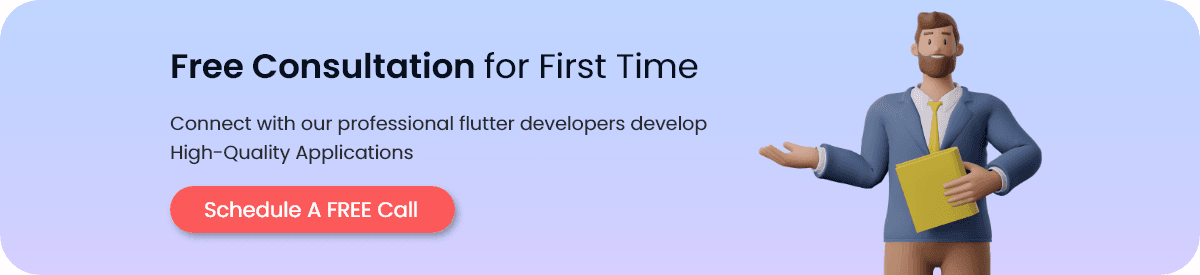
Conclusion
Hive is a no-lag and fast NoSQL database that is quite user-friendly and integrates seamlessly with Flutter. It can be used to store data locally on the device or even on the cloud. In this blog, we discussed how to use Hive in Flutter to store and retrieve data from a local database. We also covered how to update and delete data from the database, and how to close the box when we are done using it.
Using Hive in your Flutter application can improve performance by reducing the amount of network calls needed to retrieve data. Hive also offers the advantage of being able to work with data offline.
If you want to learn more about Hive, you can check out the official documentation at https://docs.hivedb.dev/.
FAQs
Hive is a lightweight, NoSQL, embedded database for Flutter. It uses Hive as a local database that stores data on the device.
Hive is a great choice for Flutter applications, as it is lightweight, fast, and easy to use. It is also highly scalable, so it can handle large amounts of data without any performance issues. Additionally, Hive supports encryption, so your data is secure.
To install Hive, add the necessary dependencies to your pubspec.yaml file and run flutter pub get to download and install them.
To use Hive in Flutter, you need to initialise Hive, register your models, open a box to store your data, and then add, retrieve, update, and delete data from the database.
Yes, Hive can be used to store data on the cloud using Hive Cloud. Hive Cloud is a cloud service offered by the Hive team that allows you to sync your local database with the cloud.