Rate Limiting Using Throttler In NestJs
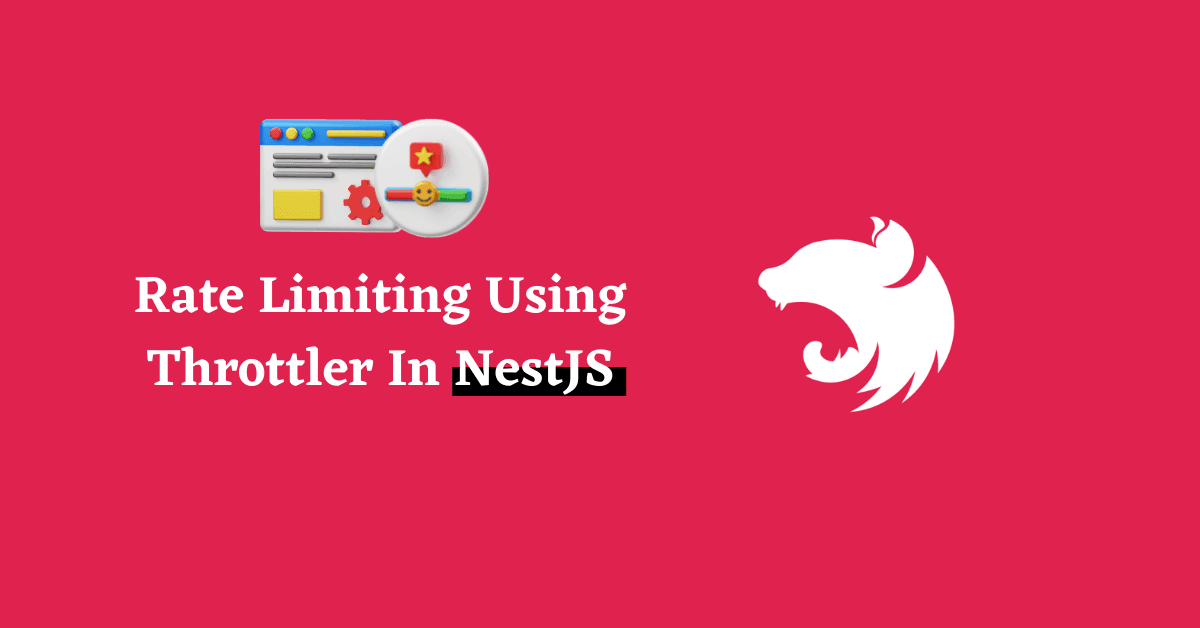
Introduction
Rate limiting is used to restrict users to hit an endpoint for a limited amount of time. In other words, by rate limiting, we can control the number of incoming requests per time. As we can define the user can hit an endpoint 10 times every minute. So, if anyone hits that endpoint more than 10 times, then it will throw an error and the user can not access that URL for a limited amount of time.
Why do We Need To Use Rate Limiting?
- Rate limiting is mainly used for managing the frequency of incoming requests per duration.
- Better server load management.
- Reduced risk of attacks like Brute Force.
- The server will never be overloaded by incoming API calls.
Drawbacks of Rate Limiting
As we discussed, Rate Limit handles the number of requests per specified duration, so in some cases, it may block our traffic from accessing our site.
Sometimes it may block legitimate users from accessing our site. It can not identify between legitimate and spam traffic. Due to this our real users might not be able to access our application.
Example of Rate Limiting in NestJs
In this example, we will see how we can use the Throttler package for rate limiting.
Step 1
Install package:
npm i --save @nestjs/throttler
Step 2
After the successful installation of the package, you can use ThrottlerModule just like any other module of the nest. You can use this module with forRoot or forRootAsync methods. In your app.module.ts file import module like :
@Module({
imports: [
ThrottlerModule.forRoot({
ttl : 60,
limit : 10,
}),
],
})
export class AppModule {}
In the above code “ttl” is Time To Live, which means the amount of time at which you want to restrict the requests. And the limit is used for the amount of requests per ttl.
Step 3
After importing you need to use a guard to use this module globally.
For that you need to do like:
providers: [
{
provide: APP_GUARD,
useClass: ThrottlerGuard,
},
]
By doing this now your Rate limit is set to 10 Requests per minute for every endpoint of your project.
app.module.ts file will look like this:
import { Module } from '@nestjs/common';
import { AppController } from './app.controller';
import { AppService } from './app.service';
import { UsersModule } from './users/users.module';
import { ThrottlerModule, ThrottlerGuard } from '@nestjs/throttler';
import { APP_GUARD } from '@nestjs/core';
@Module({
imports: [UsersModule,
ThrottlerModule.forRoot({
ttl: 60,
limit: 2
})
],
controllers:[AppController],
providers: [AppService,
{
provide: APP_GUARD,
useClass: ThrottlerGuard
}
]
})
export class AppModule {}
Read More: Migrations with TypeORM in NestJs
Step 4
Now if you want to Skip some modules from Rate limiting then you need to specify SkipThrottle() function in that controller like:
@SkipThrottle()
@Controller('users')
export class UsersController {}
Now the rate limit will not apply to any of this controller’s route.
If you want to override “ttl” or “limit” which is set globally, then you need to mention that like:
@Throttle(3, 60)
@Get('list')
@HttpCode(200)
list() {
return "List works with custom rate limiting.";
}
This will override default configurations for this specific route.
import { Controller, Get, HttpCode } from '@nestjs/common';
import { SkipThrottle, Throttle, ThrottlerGuard } from '@nestjs/throttler';
@SkipThrottle()
@Controller('users')
export class UsersController {
// This route will skip rate limiting.
@Get('list-skip')
@HttpCode(200)
listSkip(){
return "List work without Rate limiting"
}
// Rate limiting is applied to this route.
@SkipThrottle(false)
@Get('list')
@HttpCode(200)
dontSkip(){
return "List work with Rate limiting"
}
// Override default configuration for Rate limiting and duration.
@Throttle(3, 60)
@Get('get-profile')
@HttpCode(200)
getProfile(){
return "Get profile with custom Rate limiting."
}
}
Where We Can Use This Technique In Our App?
In any app, the most important thing is log in and Register APIs. So we need to secure those APIs first. So, we can apply rate limiting on the APIs like Login, Register, Forgot password, etc.
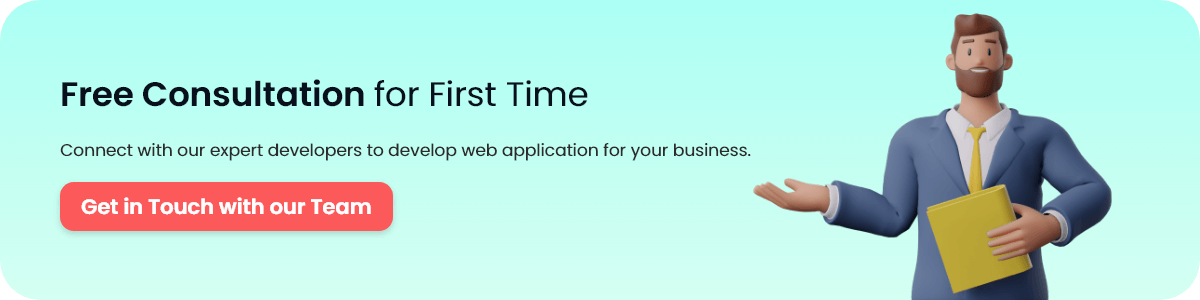
Any Payment-related API – If your APP contains a Payment-related API where you are transferring some payment data then you can apply a Rate Limit there to prevent attacks like Brute force.
APIs Which Are Having Large Data – We can use this where we have large Response data in API. By doing this we can restrict multiple API calls at the same time and it will optimize the server load.