Recoil: State Management Library in React
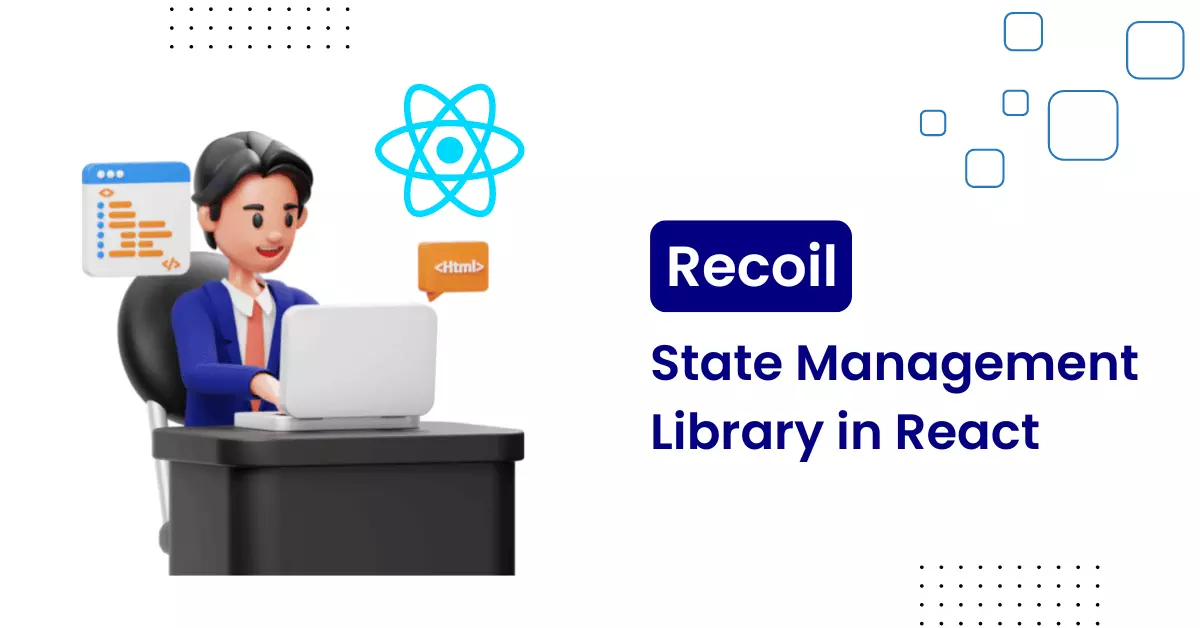
Introduction
Recoil is an open-source state management library. It provides an easy way to share the state between all components of a react application. It is very easy to set up, unlike redux which does not need boilerplate code to set up. It provides a data-graph flow that flows from atoms i.e. our shared states to selectors i.e a pure function and then into the react component.
Atoms
Just like redux, Recoil provides a global state, called Atoms. Atoms are a single source of truth that contains all states of an application. All components of our react application can access them and can subscribe to changes made on them.
Selectors
Selectors are pure functions that take atoms or other selectors as input and return output based on the changes in input.
Getting Started
npx create-react-app my-app
As Recoil is a state management library for React, First we need to create a react app.
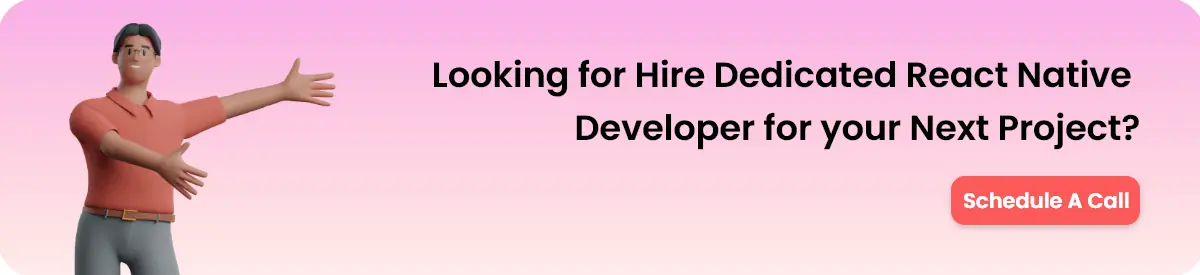
Installation
Recoil is very easy to set up. Following are the steps to get started with recoil setup in your react application:
Run the following command to install the latest stable version of Recoil if you are using npm.
npm install recoil
Or if you are using yarn then
yarn add recoil
Or if you are using bower then
bower install –save recoil
Now wrap your root component in RecoilRoot just like below
import React from "react";
import { RecoilRoot } from "recoil";
import Counter from "./counter";
function App() {
return (
<RecoilRoot>
<Counter />
</RecoilRoot>
);
}
export default App;
Now, In your counter component we will write the logic for counter application using Recoil.
First we will create an atom in our counter component that will contain the counter state.
const counterState = atom({
key: "counterState",
default: 0,
});
We have given the name counterState to our atom. A unique key counterState given to it and set the default value to 0.
useRecoilState hook is used to write and read the atom. useSetRecoilState hook is used to write to the atom while useRecoilValue hook is used to read it.
const Counter = () => {
const [counter, setCounter] = useRecoilState(counterState);
const count = useRecoilValue(countIncrementState);
const onIncrementClick = () => {
setCounter(counter + 1);
};
return (
<div className="App">
<h3>Recoil Demo</h3>
<button onClick={() => onIncrementClick()}>Increment</button>
<p>{`Current Counter State is: ${counter}`}</p>
<p>{`Next Counter State is: ${count}`}</p>
</div>
);
};
Now we have created a selector to transform the value of a state.
const countIncrementState = selector({
key: "counterIncState",
get: ({ get }) => {
return get(counterState) + 1;
},
});
After wrapping up the entire code in a file the final code will look like this
import React from "react";
import { atom, selector, useRecoilState, useRecoilValue } from "recoil";
const counterState = atom({
key: "counterState",
default: 0,
});
const countIncrementState = selector({
key: "counterIncState",
get: ({ get }) => {
return get(counterState) + 1;
},
});
const Counter = () => {
const [counter, setCounter] = useRecoilState(counterState);
const count = useRecoilValue(countIncrementState);
const onIncrementClick = () => {
setCounter(counter + 1);
};
return (
<div className="App">
<h3>Recoil Demo</h3>
<button onClick={() => onIncrementClick()}>Increment</button>
<p>{`Current Counter State is: ${counter}`}</p>
<p>{`Next Counter State is: ${count}`}</p>
</div>
);
};
export default Counter;
Below is the output for the above code.
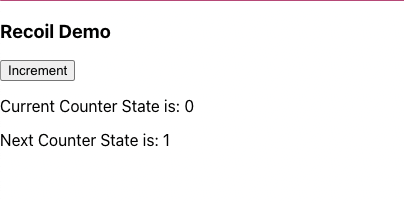
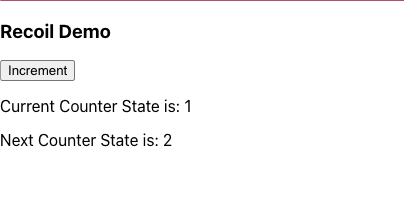
Benefits of using Recoil
- Easy to use and learn.
- Less boilerplate code.
- Data flow is simple.
- It prevents unnecessary re-renders which happen while using other state management – libraries or tools like context, redux, etc.
Cons of using Recoil
- Recoil is larger in size as compared to Redux.
- No middleware support is provided as of now.
- In Large-scaled projects, Redux is preferred over Recoil.
Conclusion
Recoil is a competent state management library. In the future, Recoil has tremendous promising potential, so if you want to use it in your large applications for state management, you won’t be disappointed. It has its own unique benefits over other state management libraries or tools. Even though Recoil is still in its early age and might take a while to establish itself, we can certainly foresee an encouraging future thanks to its easy learning curve, less boilerplate code, and elementary data flow.