How to Manage State in React Native Using Redux? – Complete Guide with Example
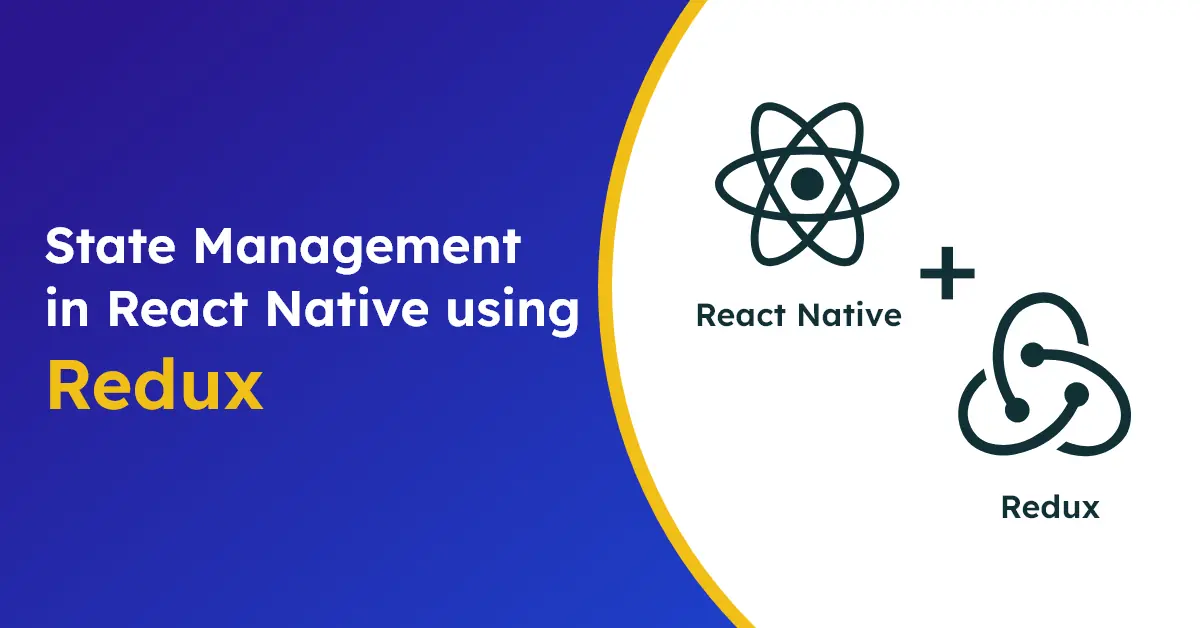
Introduction of State Management in React Native
State management is one of the most important concepts of react. Because of React’s popularity in recent times there are many state management libraries that have evolved. Redux is one of them. Redux provides an easy way to communicate between components. It provides easy debugging and a centralized store where we can manage all states of our application.
What is React Native?
React Native is an open-source JavaScript framework. It is used to create cross-platform applications. The framework allows you to create an application that can run on different platforms (e.g. Android, iOS, Android TV, tvOS, Web etc) by using the same code.
State Management in React Native
State management is one of the most key concepts in react. It is a way to communicate and share data among the components. If a user changes any state by interacting with the user interface then the UI will get updated from old state to new state.
React follows one-way data binding i.e. if any change is made in a component then data gets reflected in view. We can pass the parent component’s state to the child component by using props. But sometimes it becomes tricky when we need to share data from child component to parent component or if we want to share between sibling components. To resolve these hurdles there are many state management libraries available, one of them is redux.
State management is a crucial aspect of any application built using React Native. It allows you to manage the data flow within your app and ensure that components receive the required data to render and update correctly.
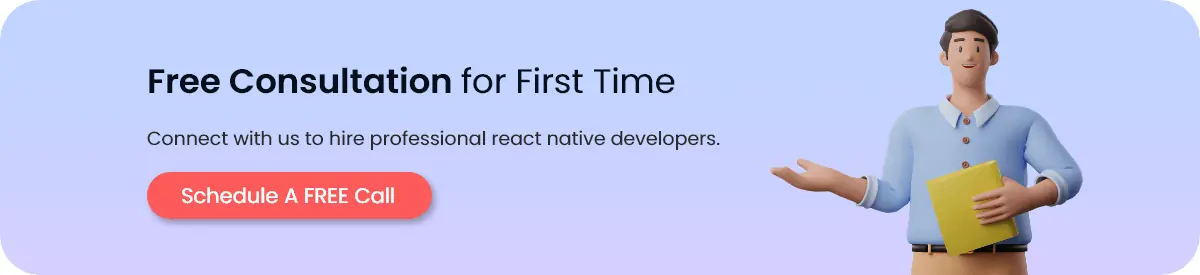
What is Redux and Why it is used in State Management?
Redux is a popular state management library that can be used with any framework. It provides a global store that contains all states of application. It is a “Single Source of Truth” which makes state management and debugging easy. In general applications, states are stored in different components of application but redux provides you a global store to manage all states of application.
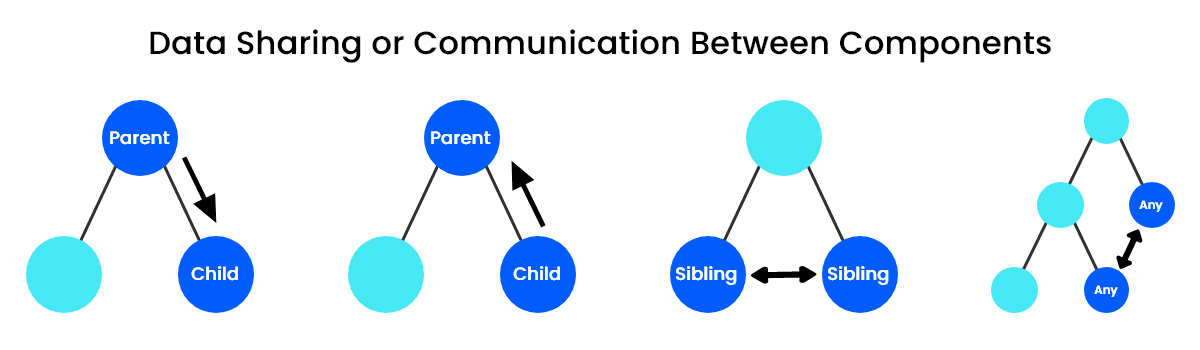
Statistics of React-Redux
The data of builtwith shows the exact data of react-redux.
- A total of 32,272 websites using react-redux which includes location information, hosting data, contact details.
- 16,055 currently live websites and an additional 57,634 domains that redirect to sites.
- 90 websites in India using react-redux.
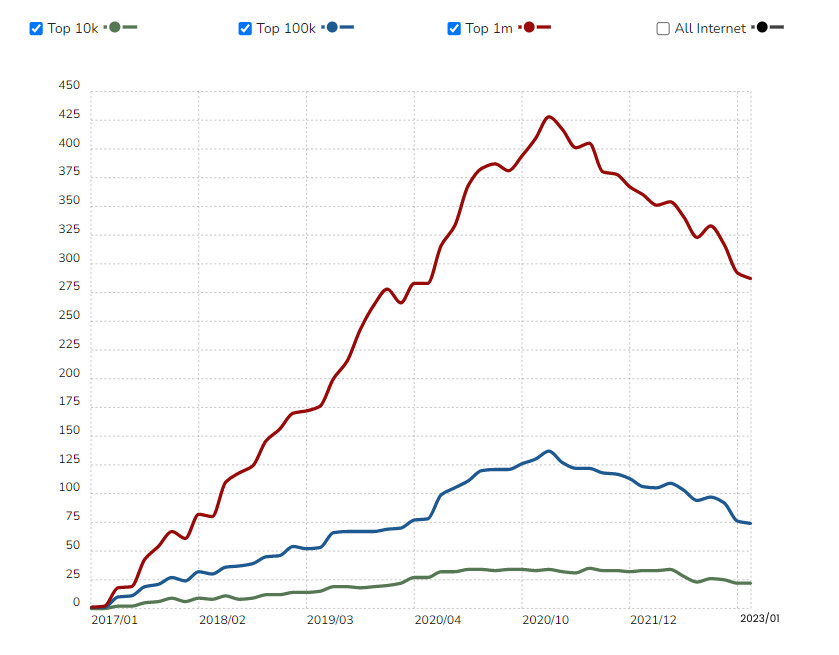
How React-Redux Works?
Add required dependencies in your package.json file:
"dependencies": {
"react" : "16.13.1",
"react-native": "0.63.2",
"react-redux": "^7.2.1",
"redux": "^4.0.5"
}
npm i react-redux
npm i redux
There is a global store that contains all states of application. Each component of the application has access to the global store.
The main working of react-redux depends on three building blocks:
- Store
- Actions
- Reducers
Create a folder named redux inside that create two more folders named as actions and reducers and create a file named as store.ts
Store: Store is a single source of truth that has entire states of application.
Example on how to configure a store: (In store.tsx)
import { createStore } from "redux"
import counterReducer from "./reducers/counterReducer"
const store = createStore(counterReducer)
export { store }
Import store in your entry point (in my demo it is app.tsx)
import React from 'react'
import { Provider } from "react-redux"
import { store } from './redux/store'
import CounterApp from './src/MainApp'
const App = () => {
return (
<Provider store={store}>
<CounterApp />
</Provider>
)
}
export default App;
Actions: Actions are nothing but events, using actions you can send data from your application to your global store i.e. Redux Store. store.dispatch() method is used to send actions. Actions are JavaScript objects that contain a type property to define the type of action and a payload property that contains information.
Example of an action :
Here we are creating a counter app which will have two actions INCREMENT and DECREMENT. So we have created two actions in counterAction.tsx file:
const incrementCounter = () => {
return {
type: "INCREMENT"
}
}
const decrementCounter = () => {
return {
type: "DECREMENT"
}
}
export { incrementCounter, decrementCounter }
Reducers: Reducers are functions that take the current state of an application and then perform any action on that and returns a new updated state.
Example of a reducer (counterReducer.tsx):
const initialState = {
counter: 0
}
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case "INCREMENT": {
return { ...state, counter: state.counter + 1 }
}
case "DECREMENT": {
return { ...state, counter: state.counter - 1 }
}
default: {
return state
}
}
}
export default counterReducer
At last we will create two buttons, one for increment and another one for decrement, where we will use dispatch() method to send our actions.
const CounterApp = () => {
const counter = useSelector(state => state.counter)
const dispatch = useDispatch()
return (
<View style={styles.container}>
<Button title="increment" onPress={() => dispatch(incrementCounter())} />
<Text>{counter}</Text>
<Button title="decrement" onPress={() => dispatch(decrementCounter())} />
</View>
)
}
Here is the final outcome:
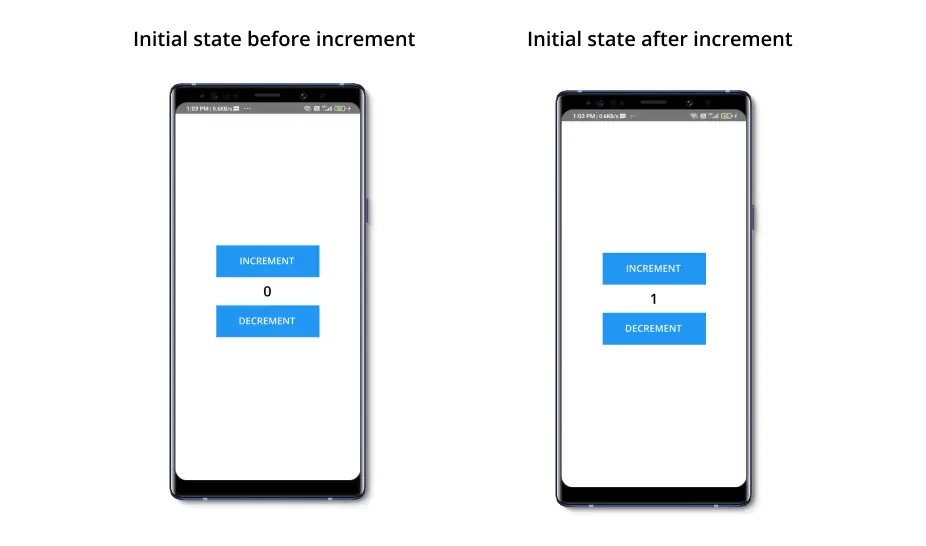
Benefits of using redux:
- Redux makes transfer of states easy between the components as we store all states in a single store. which is also known as “Single Source of Truth.”
- It makes debugging easy.
- Using redux one can write more optimized and reusable code.
- Easy to maintain.
- It can be used with any UI layer.
- Predictable state container.
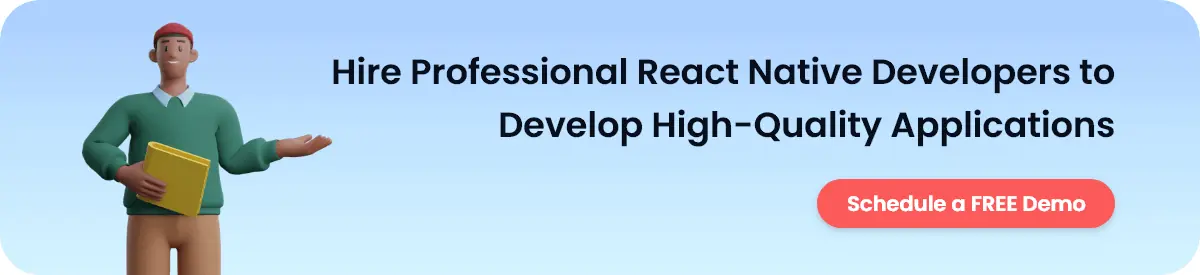
Conclusion
Redux is a very useful tool for state management in larger and complex applications. Redux provides a global store which is helpful in managing all states of an application. It makes debugging easy. Redux works with any of the UI layers which makes it different from other state management tools. If a user has a large application which needs complex state management then he/she can use redux.
FAQs
When you have too many number of states that are needed in many places then redux can be used.
Redux can be used with any framework. It is most commonly used with React and React Native but can be used with Angular, Vue.js and many more.
It’s not necessary. It is recommended to use when you have too many states in an application that needs to interact with different components of the application. It makes debugging easy.