A Complete Guide to Building a Realtime Weather App in Flutter
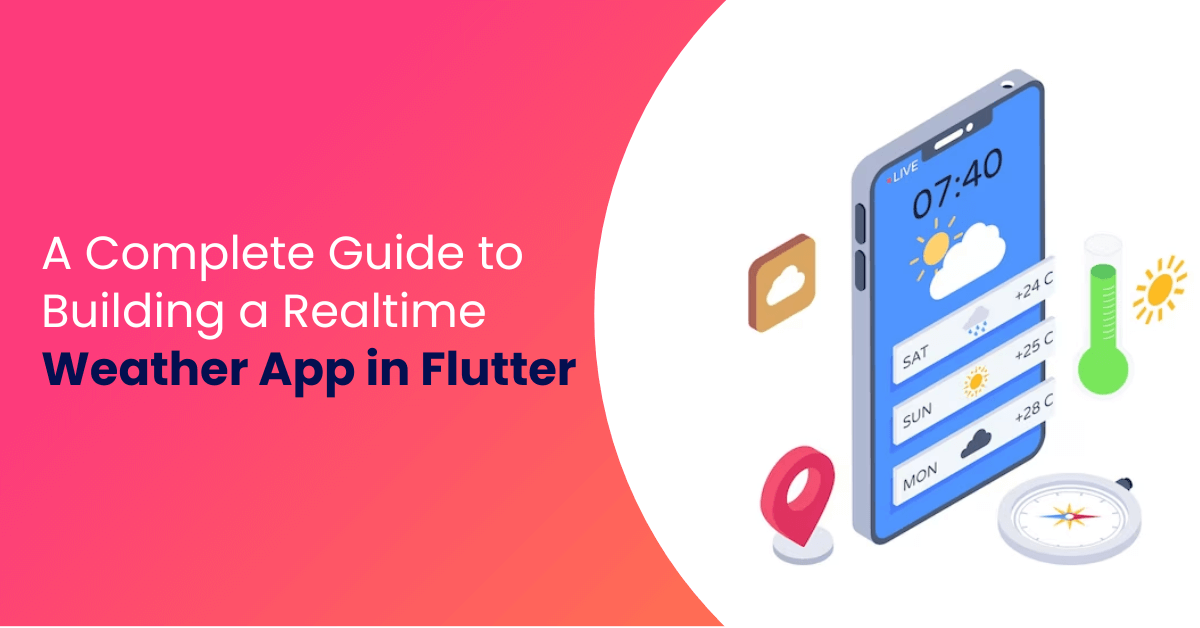
Introduction
Welcome to my first blog! I’m excited to introduce you to my simple application weather app. It provides your current location and real-time weather data, including temperature, wind speed, cloud conditions, humidity, and elevation above sea level. I built this app using the Flutter framework, which is Google’s UI toolkit. Flutter allows applications to work seamlessly on mobile, web, and desktop using a single codebase. It’s free and open source, making it a popular choice among developers and organizations worldwide.
Things We Needed
In addition to having a basic understanding of Flutter, we’ll also need access to weather-related data through an API. For this task, I opted to use OpenWeatherMap (http://api.openweathermap.org/), a reliable source that offers a free weather data API. To begin, you simply need to sign up for an account on their platform, which will provide you with a vital element: the API key. This special key is essential, as it acts as a crucial parameter needed to retrieve weather data.
Key example ===== >>>>>> 60e39g532ab04328madsccdc4g5c0ce0
Pls don’t share your key the above example is a demo key
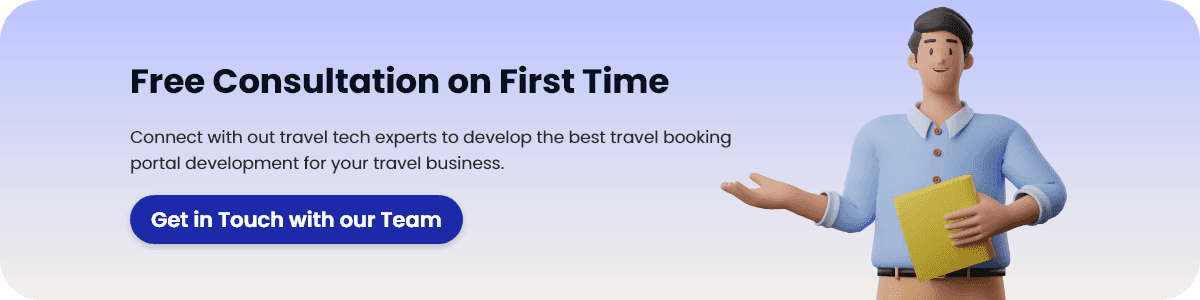
Installation required
Before a kick start all you need to install the following dependencies in your pubspec.yaml
dependencies:
flutter:
sdk: flutter
cupertino_icons: ^1.0.6
geocoding: ^2.1.1
geolocator: ^10.1.0
http: ^1.1.0
sleek_circular_slider: ^2.0.1
get: ^4.6.5
permission_handler: ^11.0.1
intl: ^0.18.1
In the dependencies mentioned above, ‘geolocator’ and ‘geocoding’ are essential, while others can vary depending on your project requirements. For example, in my project, I utilized the ‘getx’ library, so I installed the ‘get’ dependency accordingly.
Add these dependencies in your Androidmanifest.xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.weatherapp_starter_project">
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_BACKGROUND_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.INTERNET" />
Coding part
How to access the location using geolocator and geocoding
@override
void onInit() {
initData();
super.onInit();}
initData() async {
if (_isLoading.isTrue) {
await getLocation();
await getAddress(_latitude.value, _longitude.value);
}
}
getLocation() async {
bool isServiceEnabled;
LocationPermission locationPermission;
await Geolocator.requestPermission();
isServiceEnabled = await Geolocator.isLocationServiceEnabled();
if (!isServiceEnabled) {
return Future.error('Location not enabled');
}
locationPermission = await Geolocator.checkPermission();
if (locationPermission == LocationPermission.deniedForever) {
return Future.error('Location permission are denied forever');
} else if (locationPermission == LocationPermission.denied) {
if (locationPermission == LocationPermission.denied) {
return Future.error('Location permission is denied');
}
}
return await Geolocator.getCurrentPosition(
desiredAccuracy: LocationAccuracy.high)
.then((value) {
_latitude.value = value.latitude;
_longitude.value = value.longitude;
_isLoading.value = false;
weatherController.weather(_latitude.value, _longitude.value);
});
}
Future<void> getAddress(double lat, double lon) async {
List<Placemark> placemarks = await placemarkFromCoordinates(lat, lon);
Placemark place = placemarks[0];
city.value = place.locality!;
}
How to call an API using with longitude and latitude as a parameter which we get from above code
Future<WeatherModel?> weather(double lat, double lon) async {
final WeatherService weatherService = WeatherService();
final APIResponse response = await weatherService.weather(lat, lon);
if (response.ok) {
final Map<String, dynamic> responseData = json.decode(response.data);
print('response>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>$responseData');
final WeatherModel weatherModel = WeatherModel.fromJson(responseData);
currentTemperature.value = weatherModel.main?.temp ?? 0.0;
iconCode.value = weatherModel.weather?.first.icon ?? '';
winds.peed.value = weatherModel.wind?.speed ?? 0.0;
clouds.value = weatherModel.clouds?.all ?? 0;
humidity.value = weatherModel.main?.humidity ?? 0;
maxTemp.value = weatherModel.main?.tempMax ?? 0.0;
minTemp.value = weatherModel.main?.tempMin ?? 0.0;
} else {
return null;
}
return null;
}
Service class
class WeatherService {
Future<APIResponse> weather(double lat, double lon) async {
try {
String url = '${APIKey.baseUrl}/data/2.5/weather?lat=$lat&lon=$lon&appid=90d29f532bb0 4328dadcccdc4f5c0ce0&units=metric';
APIResponse response = await Http().handleHttpRequest<APIResponse>(
"Unable to find data",
() async => await Http.get(url),
);
return response;
} catch (e) {
return APIResponse(
false,
data: e.toString(),
message: "Error in finding data.",
Severity: ErrorLevel.Connection,
);
}
}
Conclusion
Our weather app is ready, providing you with real-time information about your current location, including temperature, humidity, wind speed, and other relevant details tailored to your preferences
FAQ
The OpenWeatherMap API provides different endpoints based on your needs. For example, you can use the city name endpoint or the latitude and longitude endpoint to access weather data.
No, you don’t need to subscribe. Simply create an account, and a unique token key will be generated for you. The free API access is available for OpenWeatherMap version 2.5. However, for the latest version 3.0, there is a fee.
Answer: The recent API response no longer includes hourly, minutely, and daily data for free. These features are now part of a paid subscription.